Send Email using Amazon SES in PHP
First of all, you need to install the AWS SDK for PHP. We need to install the composer(you can get it from the getcomposer.org) in our project then we need to run the below command to install the AWS SDK in our project.
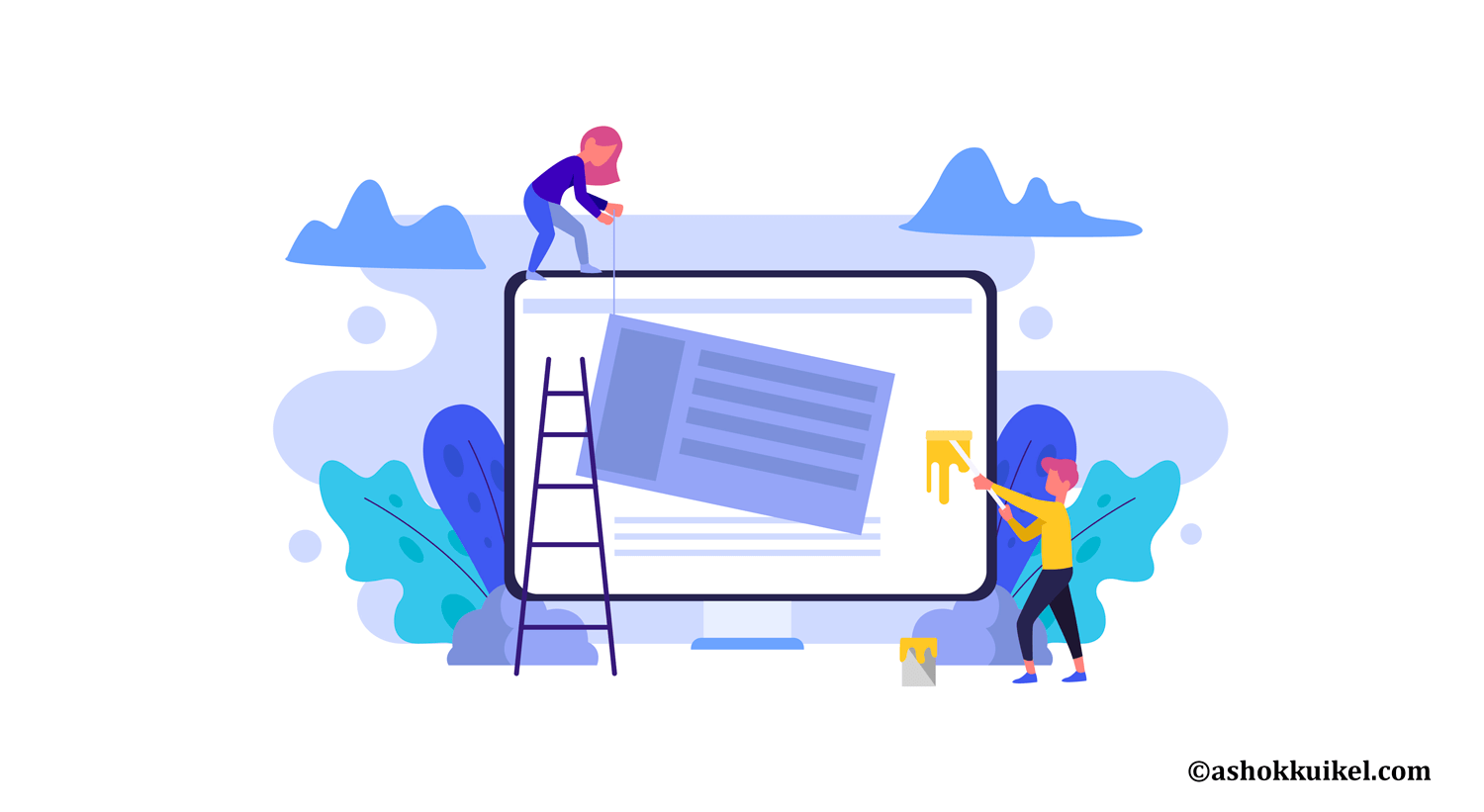
In this article, we have discussed how to send amazon SES(Simple Email Service) mail using PHP. First, you need to install the AWS SDK for PHP. For that first, we need to install the composer(you can get from the getcomposer.org) in our project then after we need to run the below command to install the AWS SDK in our project.
composer require aws/aws-sdk-php
After installing the AWS SDK in our project we need to “autoload.php” file in our root project or specific location where we need to use these AWS SDK services. For that, we need to add
require ‘vendor/autoload.php’;
In our project. after that, we can use AWS SDK in our project where we can need it. Here we do code for the Amazon SES API to send an email. let’s create email.php file for sending email,
// If necessary, modify the path in the require statement below to refer to the
// location of your Composer autoload.php file.
require ‘vendor/autoload.php’;use Aws\Ses\SesClient;
use Aws\Exception\AwsException;
after adding the above code we need a key and secret key from amazon. You can get from the Amazon create IAM User from that you can get these detail.
$key = ‘####’;
$secret = ‘###’;
$SesClient = new SesClient([
‘version’ => ‘latest’,
‘region’ => ‘us-east-1’,
“Statement” => [
“Effect” => “Allow”,
“Action” => [
“ses” => “*”
],
“Resource” => “*”
],
‘credentials’ => [
‘key’ => $key,
‘secret’ => $secret,
]
]);
In the above code, we have initialized the SESClient class with our values. Change region according to your profile.
//Sender email address verified in Amazon SES where we setup.
// This address must be verified with Amazon SES.
$sender_email = ‘[email protected]’;// Replace these sample addresses with the addresses of your recipients.
$recipient_emails = [‘[email protected]’];$subject = ‘Test Amazon SES ‘;
$plaintext_body = ‘This email was sent with AWS SDK for PHP.’ ;
$html_body = ‘<h1>AWS Amazon Simple Email Service Test Email</h1>’.
‘<p>First email Amozon SES.</p>’;
$char_set = ‘UTF-8’;try {
$result = $SesClient->sendEmail([
‘Destination’ => [
‘ToAddresses’ => $recipient_emails,
],
‘ReplyToAddresses’ => [$sender_email],
‘Source’ => $sender_email,
‘Message’ => [
‘Body’ => [
‘Html’ => [
‘Charset’ => $char_set,
‘Data’ => $html_body,
],
‘Text’ => [
‘Charset’ => $char_set,
‘Data’ => $plaintext_body,
],
],
‘Subject’ => [
‘Charset’ => $char_set,
‘Data’ => $subject,
],
],
]);
$messageId = $result[‘MessageId’];
echo(“Email sent! Message ID: $messageId”.”\n”);
} catch (AwsException $e) {
// output error message if fails
echo $e->getMessage();
echo(“The email was not sent. Error message: “.$e->getAwsErrorMessage().”\n”);
echo “\n”;
}
Leave a Reply
You must be logged in to post a comment.